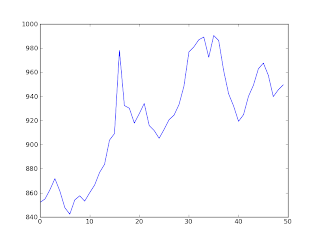
Around May 2011, the time I decided to go on solo bike rides and GoogleMaps has been of great help ever since. It was only after a few months, I realized there was a python binding for GoogleMaps. Only after going on an unplanned solo cycling trip recently, I realized how much of a help it can be. Since most of the times I'm on highways and well raid roads, it is possible to get the elevation of the whole route. When the elevations are plotted, it gives a vague idea of the terrain. On the left the terrain of road from Channarayapatna to Hassan (Elevation is in mtrs).
Enough talk and here is the code. The elevation api has been used to get elevation of a point and googlemaps api has been used to get the latitude and longitude of start and end points. The points along the route have been interpolated as of now. But they can be obtained point by point using googlemaps api and interpolated based on the distance.
Will probably do all that and make another post soon. Happy coding till then.